
SingleStore April Challenge: Haiku ASCII
Notebook

📣 SingleStore's Creative Challenge for #NationalPoetryMonth: Win RayBan Smart sunglasses 😎 and a $500 AWS gift card! 💸
This notebook can be run on the SingleStore Free Shared Tier Workspace.
To create a Shared Tier Workspace, please visit: https://www.singlestore.com/cloud-trial
🎉 All throughout April, SingleStore is hosting a challenge inviting participants to craft a unique Haiku or create captivating ASCII art using SingleStore Notebooks. The most creative masterpiece wins a set of Meta RayBan Smart sunglasses and a $500 AWS gift card!
➡️ Steps to Participate
✅ Activate your Free Shared Tier with SingleStore here: https://www.singlestore.com/cloud-trial
✅ Create your artistic masterpiece in a SingleStore Notebook, drawing inspiration from this example Notebook and your unique vision.
✅ Share a Github link to your notebook by April 30, 2024: https://docs.google.com/forms/d/e/1FAIpQLSdXcvzSxtTtHYxRG40Pc5HVknxu6EbngDrsX6ukzkEbRu26ww/viewform
✅ Make sure to tag @SingleStore and use #SingleStorePoetry when sharing your work on LinkedIn/X
For questions about this contest or SingleStore Notebooks, use our dedicated Discord channel: https://discord.gg/re56Fwyd
Feel free to make changes to this starter code to generate a Haiku and ASCII art. The below code consists of two main parts:
Generating a Haiku: The generate_haiku function creates a simple haiku using pre-defined lists of phrases that correspond to the traditional 5-7-5 syllable structure of haikus. This function randomly selects one phrase from each list to construct the haiku.
Visualizing the Haiku: The visualize_haiku function uses matplotlib to create a visualization of the generated haiku. It sets up a figure with a custom background color, hides the axes for a cleaner look, and displays the haiku text in the center with a styled bounding box around it.
In [1]:
!pip install matplotlib --quiet
Generating a Haiku with Seasonal Transitions
In [2]:
import numpy as npimport matplotlib.pyplot as pltfrom matplotlib.colors import LinearSegmentedColormapimport matplotlib.image as mpimg # Import for loading the logo imageimport requests # Import requests to download the logofrom PIL import Image # Import PIL to handle the imagefrom io import BytesIO # Import BytesIO to handle the image as a byte stream# Seasonal Transition Haiku Generatordef generate_seasonal_transition_haiku():seasons = ["Winter", "Spring", "Summer", "Fall"]transitions = {("Winter", "Spring"): ["Data thaws with grace,", # 5 syllables"Queries swiftly bloom, unfurl,", # 7 syllables"Insight crisp and clear." # 5 syllables],("Spring", "Summer"): ["Insights grow in light,", # 5 syllables"Data scales the bright, warm sun,", # 7 syllables"Wisdom on full show." # 5 syllables],("Summer", "Fall"): ["Leaves of data turn,", # 5 syllables"Decisions ripe and well-earned,", # 7 syllables"Change whispers anew." # 5 syllables],("Fall", "Winter"): ["Data's frost sets in,", # 5 syllables"Vector cycles glean insights,", # 7 syllables"Silence holds the keys." # 5 syllables],}# Randomly select a transitionstart_season = np.random.choice(seasons)end_season = seasons[(seasons.index(start_season) + 1) % len(seasons)]haiku_lines = transitions[(start_season, end_season)]return haiku_lines, start_season, end_season# Visualization with Gradientdef visualize_seasonal_transition(haiku, start_season, end_season):fig, ax = plt.subplots(figsize=(10, 6))ax.axis('off')# Seasonal Colorscolors = {"Winter": "#ffffff","Spring": "#4caf50","Summer": "#ffa726","Fall": "#fb8c00"}# Create Gradientcmap = LinearSegmentedColormap.from_list("season_transition", [colors[start_season], colors[end_season]])gradient = np.linspace(0, 1, 256)gradient = np.vstack((gradient, gradient))ax.imshow(gradient, aspect='auto', cmap=cmap, extent=[0, 10, 0, 10])# Display Haikuax.text(5, 5, "\n".join(haiku), ha='center', va='center', fontsize=14, color="black")# Download the logologo_url = "https://raw.githubusercontent.com/singlestore-labs/spaces-notebooks/master/common/images/singlestore-logo-vertical.png"response = requests.get(logo_url)logo_img = Image.open(BytesIO(response.content))# Convert the Image object to a NumPy array and display itlogo = np.array(logo_img)ax_logo = fig.add_axes([0.75, -0.05, 0.15, 0.15]) # Adjust these values to position and scale the logoax_logo.imshow(logo)ax_logo.axis('off') # Hide the axis of the logo subplotplt.show()# Generate and Visualizehaiku, start_season, end_season = generate_seasonal_transition_haiku()visualize_seasonal_transition(haiku, start_season, end_season)
ASCII Art Generation
Note that you might have to add the URL to firewall when asked to do so, to be able to access your input image in the below code
In [3]:
!pip install Pillow requests --quiet
In [4]:
import requestsfrom PIL import Imagefrom io import BytesIO# Define the ASCII characters that will be used to replace the pixels.ASCII_CHARS = ["@", "#", "S", "%", "?", "*", "+", ";", ":", ",", "."]def resize_image(image, new_width=100):width, height = image.sizeratio = height / width / 1.65 # Correct for aspect rationew_height = int(new_width * ratio)resized_image = image.resize((new_width, new_height))return resized_imagedef grayify(image):grayscale_image = image.convert("L")return grayscale_imagedef pixels_to_ascii(image):pixels = image.getdata()ascii_str = ''for pixel in pixels:ascii_str += ASCII_CHARS[pixel // 25] # Map the pixel value to ASCII_CHARSreturn ascii_strdef process_image_from_url(image_url, new_width=100):# Fetch the image from the URLresponse = requests.get(image_url)if response.status_code == 200:# Open the image from the bytes in response contentimage = Image.open(BytesIO(response.content))# Process the imageimage = resize_image(image, new_width)image = grayify(image)ascii_str = pixels_to_ascii(image)# Format the ASCII string so that each line has `new_width` characters.img_width = image.widthascii_str_len = len(ascii_str)ascii_img = ""for i in range(0, ascii_str_len, img_width):ascii_img += ascii_str[i:i+img_width] + "\n"# Print the ASCII artprint(ascii_img)else:print("Failed to retrieve the image from the URL")# Example usage with a public image URLimage_url = 'https://raw.githubusercontent.com/singlestore-labs/spaces-notebooks/master/common/images/singlestore-banner.png' # Replace with your image's URLprocess_image_from_url(image_url, 100)
➡️ Next Steps:
✅ Share a Github link to your notebook by April 30, 2024: https://docs.google.com/forms/d/e/1FAIpQLSdXcvzSxtTtHYxRG40Pc5HVknxu6EbngDrsX6ukzkEbRu26ww/viewform
✅ Make sure to tag @SingleStore and use #SingleStorePoetry when sharing your work on LinkedIn/X
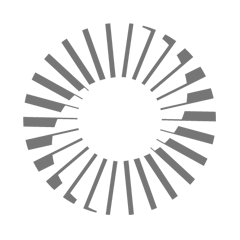
Details
About this Template
SingleStore is hosting a challenge inviting participants to craft a unique Haiku or create captivating ASCII art using SingleStore Notebooks. The most creative masterpiece wins a set of Meta RayBan Smart sunglasses and a $500 AWS gift card!"
Tags
License
This Notebook has been released under the Apache 2.0 open source license.