Wondering what LangChain is and how it works? Check out this absolute beginner's guide to LangChain, where we discuss what LangChain is, how it works, the prompt templates and how to build applications using a LangChain LLM.
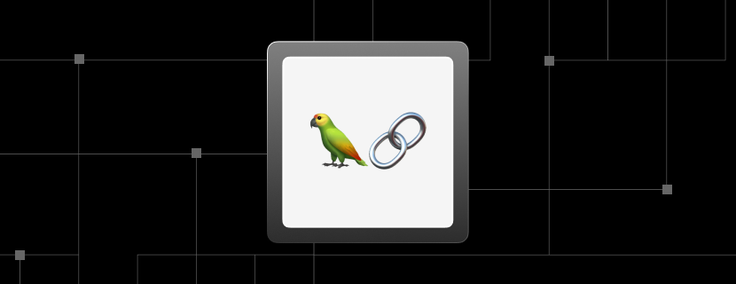
In an era where artificial intelligence is reshaping the boundaries of possibility, LangChain emerges as a powerful framework designed to leverage the capabilities of Large Language Models (LLMs). At the intersection of innovation and practicality, LangChain offers a toolkit that allows developers to chain together AI language capabilities, creating complex applications with unprecedented ease.
In this article, we will delve into the intricacies of LangChain and understand how it simplifies the process of building AI-driven language solutions. Whether you're a seasoned developer or an AI enthusiast keen to learn about the latest advancements, join us as we decode the mechanics behind LangChain — and reveal the potential it holds for the future of AI interactions.
What is LangChain?
Developed by Harrison Chase and debuted in October 2022, LangChain serves as an open-source platform designed for constructing sturdy applications powered by LLMs, such as chatbots like ChatGPT and various tailor-made applications.
LangChain seeks to equip data engineers with an all-encompassing toolkit for utilizing LLMs in diverse use cases, including chatbots, automated question answering, text summarization and beyond.
LangChain is composed of six key modules:
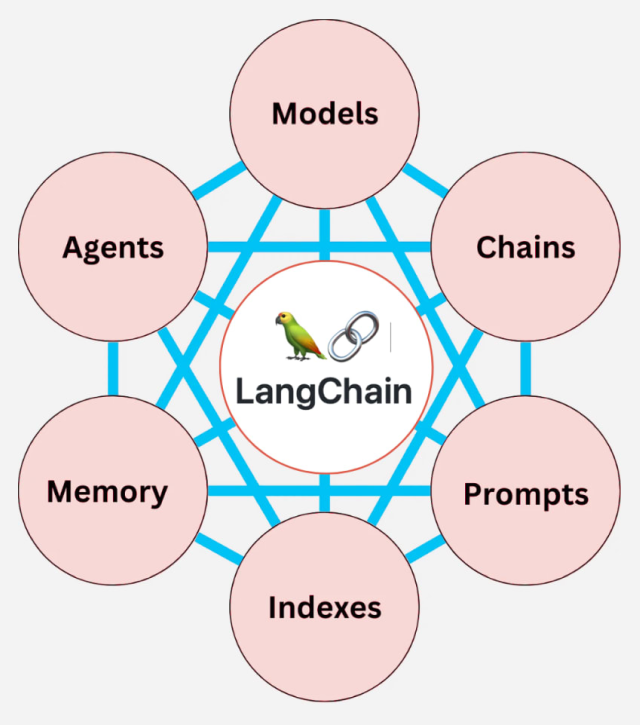
Image credits: ByteByteGo
LLMs. LangChain serves as a standard interface that allows for interactions with a wide range of LLMs.
- Prompt construction. LangChain offers a variety of classes and functions designed to simplify the process of creating and handling prompts.
- Conversational memory. LangChain incorporates memory modules that enable the management and alteration of past chat conversations, a key feature for chatbots that need to recall previous interactions.
- Intelligent agents. LangChain equips agents with a comprehensive toolkit. These agents can choose which tools to utilize based on user input.
- Indexes. Indexes in LangChain are methods for organizing documents in a manner that facilitates effective interaction with LLMs.
- Chain. While using a single LLM may be sufficient for simpler tasks, LangChain provides a standard interface and some commonly used implementations for chaining LLMs together for more complex applications, either among themselves or with other specialized modules.
How does LangChain work?
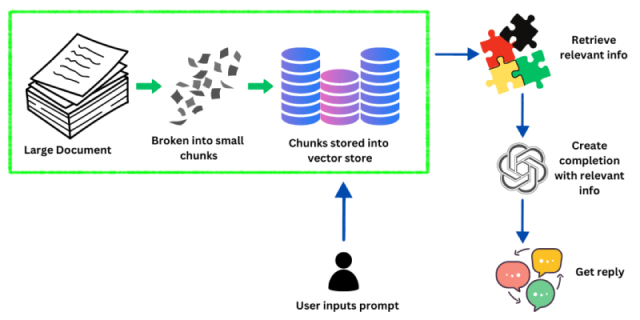
The preceding image shows how LangChain handles and processes information to respond to user prompts. Initially, the system starts with a large document containing a vast array of data. This document is then broken down into smaller, more manageable chunks.
These chunks are subsequently embedded into vectors — a process that transforms the data into a format that can be quickly and efficiently retrieved by the system. These vectors are stored in a vector store, essentially a database optimized for handling vectorized data.
When a user inputs a prompt into the system, LangChain queries this vector store to find information that closely matches or is relevant to the user's request. The system employs large LLMs to understand the context and intent of the user's prompt, which guides the retrieval of pertinent information from the vector store.
Once the relevant information is identified, the LLM uses it to generate or complete an answer that accurately addresses the query. This final step culminates in the user receiving a tailored response, which is the output of the system's data processing and language generation capabilities.
LangChain tutorial: Get started with LangChain
Let's use SingleStore's Notebooks feature (it is free to use) as our development environment for this tutorial.
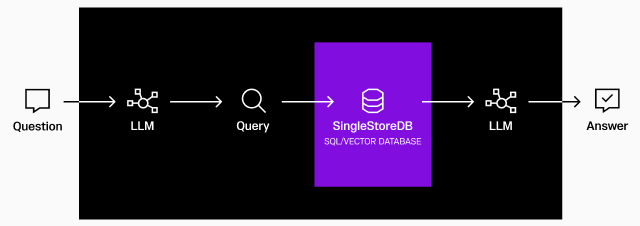
The SingleStore Notebook extends the capabilities of Jupyter Notebook to enable data professionals to easily work and play around.
Signup for SingleStoreDB to use Notebooks.
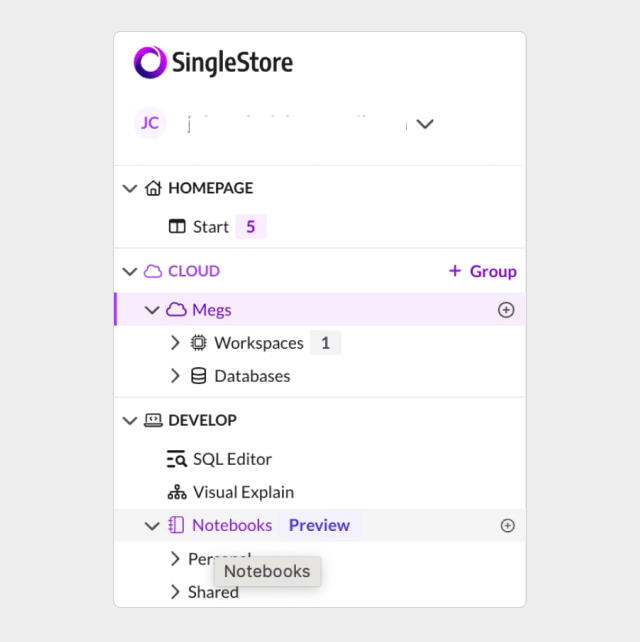
Once you sign up, you will also receive $60 in free computing resources — so why not use this opportunity?
Click on 'Notebooks' and start with a blank Notebook.
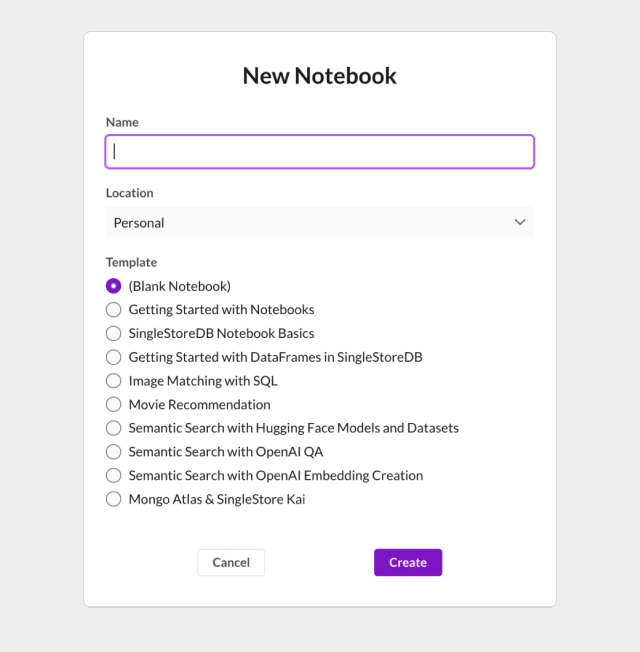
Name it something like 'LangChain-Tutorial,' or anything else that works for you.
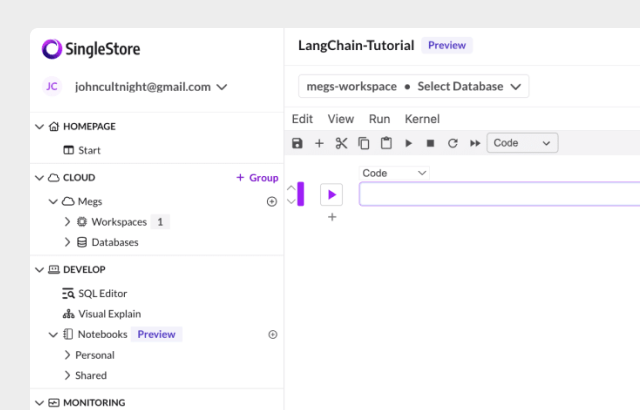
Let's start working with our Notebook that we just created.
Follow this step-by-step guide and keep adding the code shown in each step in your Notebook and execute it. Let's start!
Now, to use Langchain, let’s first install it with the pip command.
!pip install -q langchain
To work with LangChain, you need integrations with one or more model providers like OpenAI or Hugging Face. In this example, we’ll use OpenAI’s APIs. Let’s install it.
!pip install -q openai
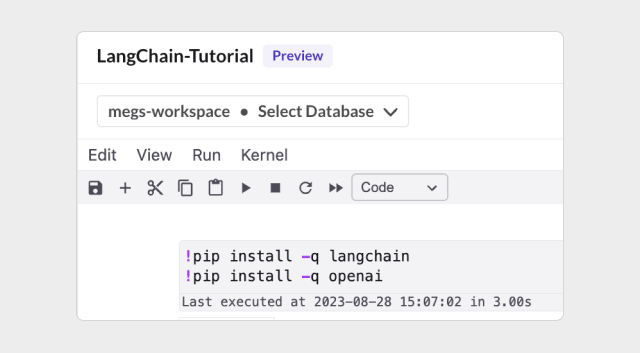
Next, we need to set up the environment variable to play around. Let's do that.
import os
os.environ["OPENAI_API_KEY"] = "Your-API-Key"
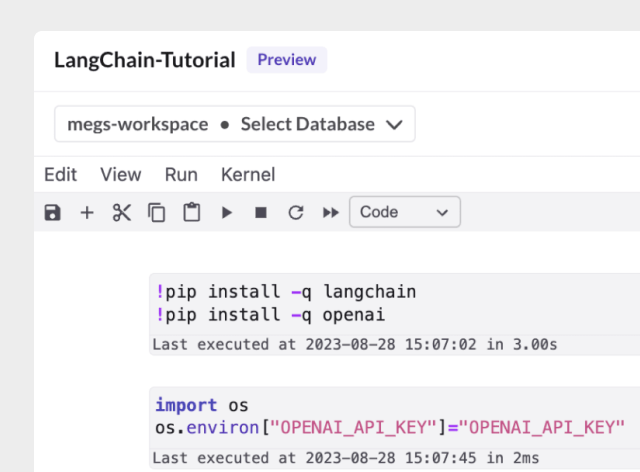
You’ll need to know how to get your API key. If you don’t, log in to your OpenAI account and follow the instructions listed.
[Note: Make sure you still have the quota to use your API key]
Next, let’s get an LLM like OpenAI and predict with this model. We’ll ask our model to tell us the top five most populated cities in the world.
from langchain.llms import OpenAI
llm = OpenAI(temperature=0.7)
text = "what are the 5 most populated cities in the world?"
print(llm(text))
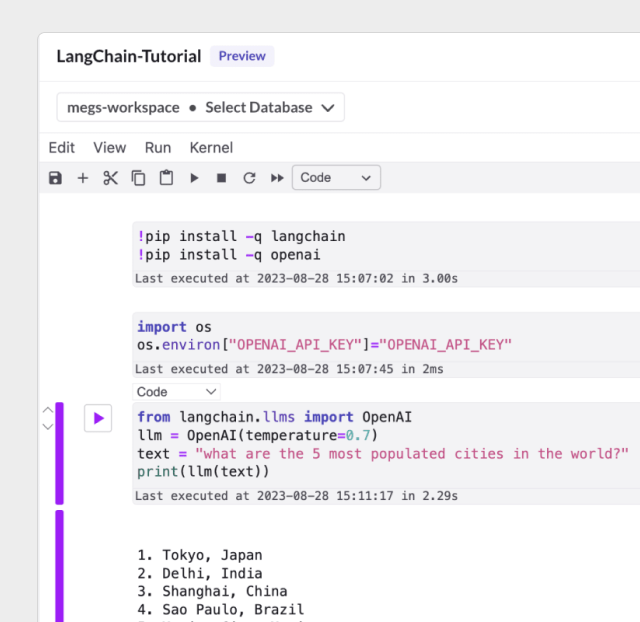
As you can see, our model made a prediction and gave us the top five cities based on population.
LangChain prompt templates
Let’s first define the prompt template.
from langchain.prompts import PromptTemplate
# Creating a prompt
prompt = PromptTemplate(
input_variables=["input"],
template="what are the 5 most {input} cities in the world?",
)
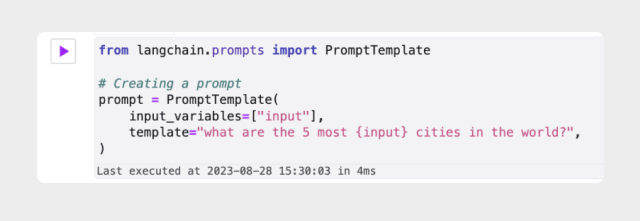
We created our prompt. To get a prediction, let’s now call the format method and pass it an input.
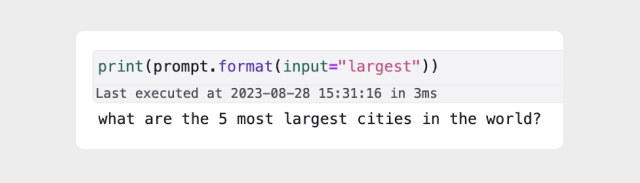
Creating chains
So far, we’ve seen how to initialize a LLM model, and how to get a prediction with this model. Now, let’s take a step forward and chain these steps using the LLMChain class.
from langchain.chains import LLMChain
# Instancing a LLM model
llm = OpenAI(temperature=0.7)
# Creating a prompt
prompt = PromptTemplate(
input_variables=["attribute"],
template= "What is the largest {attribute} in the world?",
)
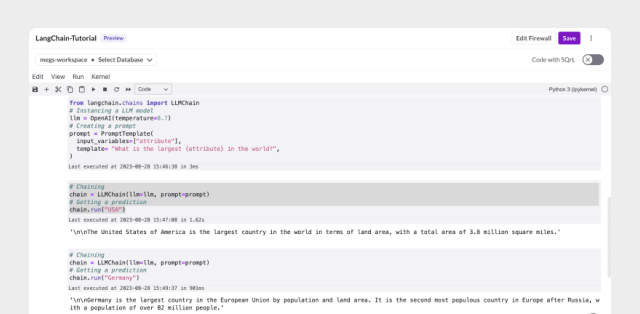
You can see the prediction of the model.
Developing an application using a LangChain LLM
Again, you’ll use SingleStore Notebooks as the development environment. Let's develop a very simple chat application.
Start with a blank Notebook and name it whatever you’d like. First, install the dependencies.
pip install langchain openai
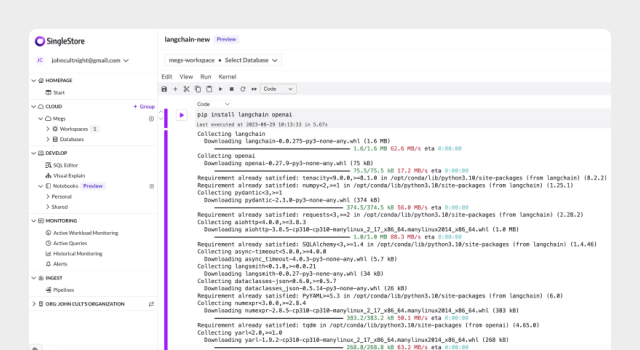
Next, import the installed dependencies.
from langchain import ConversationChain, OpenAI, PromptTemplate, LLMChain
from langchain.memory import ConversationBufferWindowMemory
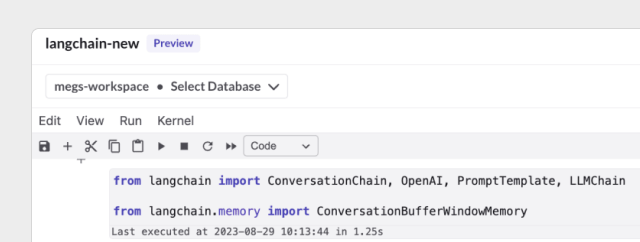
Get your OpenAI API key and save it.
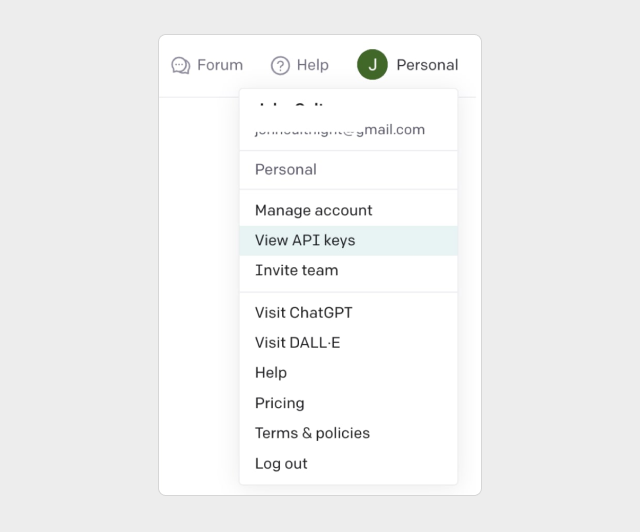
Add and customize the LLM template
# Customize the LLM template
template = """Assistant is a large language model trained by OpenAI.
{history}
Human: {human_input}
Assistant:"""
prompt = PromptTemplate(input_variables=["history", "human_input"],
template=template)
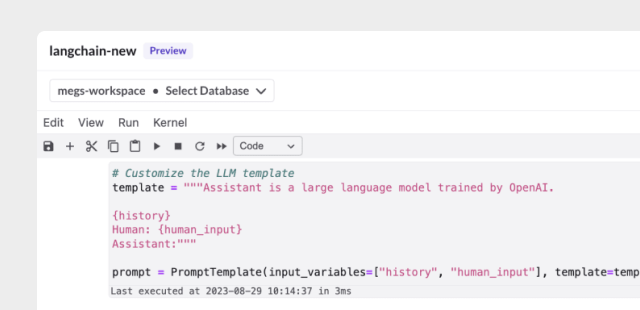
Load the ChatGPT chain with your API key you saved. Add the human input as 'What is SingleStore?'. You can change your input to whatever you want.
chatgpt_chain = LLMChain(
llm=OpenAI(openai_api_key="YOUR-API-KEY",temperature=0),
prompt=prompt,
verbose=True,
memory=ConversationBufferWindowMemory(k=2),
)
# Predict a sentence using the chatgpt chain
output = chatgpt_chain.predict(
human_input="What is SingleStore?"
)
# Display the model's response
print(output)
The script initializes the LLM chain using the OpenAI API key and a preset prompt. It then takes user input and shows the resulting output:
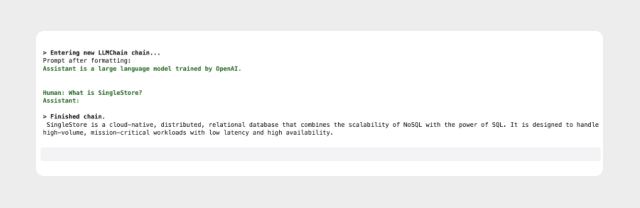
Play with this by changing the human input text/content. The complete execution steps in code format is available on GitHub. By the way, SingleStore has a fine integration with LangChain.
LangChain emerges as an indispensable framework for data engineers and developers striving to build cutting-edge applications powered by LLMs. Unlike traditional tools and platforms, LangChain offers a more robust and versatile framework tailored for complex AI applications.
LangChain is not just another tool in a developer's arsenal; it's a transformative framework that redefines what is possible in the realm of AI-powered applications.
We also sat down with LangChain CEO Harrison Chase at our real-time AI conference, SingleStore Now. You can watch our Q+A session with him on demand here.
Reap the benefits of both the worlds, using SingleStore and LangChain to make smart and powerful AI applications today.
Build AI-powered applications using SingleStore and LangChain. Start your free Singlestore Helios trial today.